Note
Go to the end to download the full example code.
Mesh generation#
This example shows how to generate a mesh from a CAD model. The CAD model is imported from a file, and the mesh is generated using the Ansys PRIME API.
import os
from pathlib import Path
from ansys.meshing import prime
from ansys.meshing.prime.graphics import Graphics
Parameters for the script#
The following parameters are used to control the script execution. You can modify these parameters to suit your needs.
GRAPHICS_BOOL = False # Set to True to display the graphics
OUTPUT_DIR = Path(Path(__file__).parent, "outputs") # Output directory
Start a PRIME session#
Start a PRIME session and get the model from the client.
prime_client = prime.launch_prime(timeout=120)
print(prime_client)
# Get the model from the client
model = prime_client.model
Using Ansys Prime Server from container ansys-prime-server-1
<ansys.meshing.prime.internals.client.Client object at 0x7f5c94fd07a0>
Load the CAD file#
Load the CAD file from the previous example. The file is loaded into the model, and the part is extracted. The part is then summarized to get the details of the part.
# Load the FMD file
modeling_file = Path(OUTPUT_DIR, "modeling_demo.fmd")
file_io = prime.FileIO(model)
file_io.import_cad(
file_name=modeling_file,
params=prime.ImportCadParams(
model=model,
),
)
# Review the part
part = model.get_part_by_name("modelingdemo")
part_summary_res = part.get_summary(prime.PartSummaryParams(model, print_mesh=False))
print(part_summary_res)
if GRAPHICS_BOOL:
display = Graphics(model=model)
display()
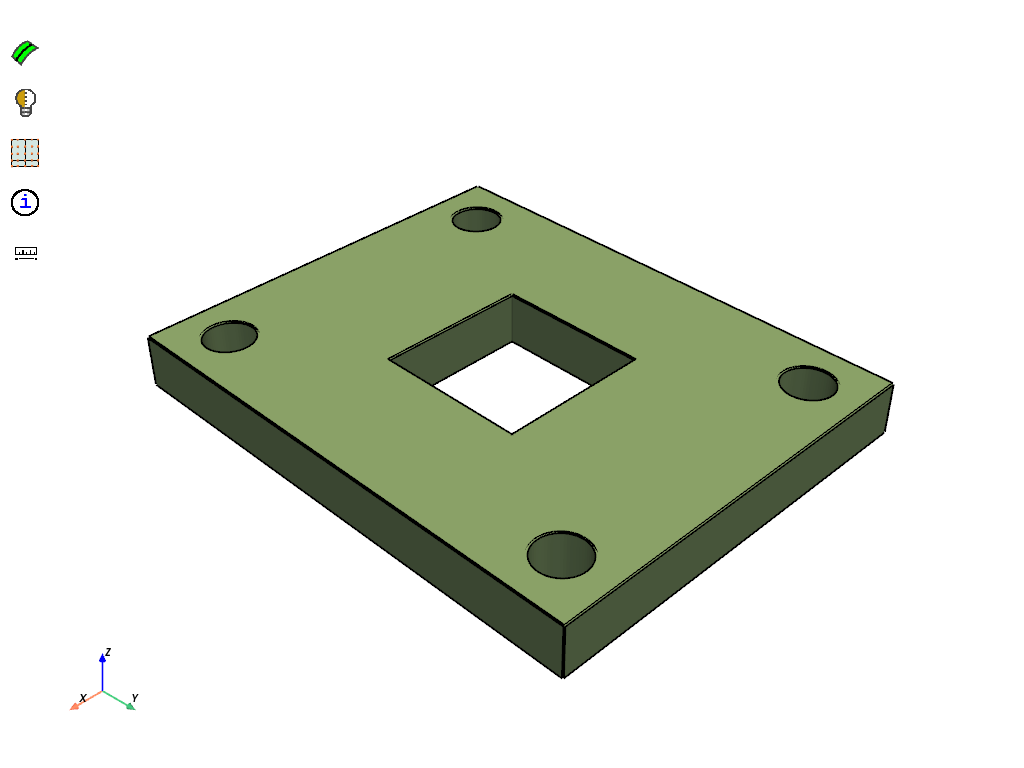
message :
Part Name: modelingdemo
Part ID: 2
32 Topo Edges
14 Topo Faces
1 Topo Volumes
0 Edge Zones
Edge Zone Name(s) : []
0 Face Zones
Face Zone Name(s) : []
1 Volume Zones
Volume Zone Name(s) : [design_body]
0 Label(s)
Names: []
Bounding box (-40 -50 0)
(40 50 10)
n_topo_edges : 32
n_topo_faces : 14
n_topo_volumes : 1
n_edge_zonelets : 0
n_face_zonelets : 0
n_cell_zonelets : 0
n_edge_zones : 0
n_face_zones : 0
n_volume_zones : 1
n_labels : 0
n_nodes : 0
n_faces : 0
n_cells : 0
n_tri_faces : 0
n_poly_faces : 0
n_quad_faces : 0
n_second_order_tri_faces : 0
n_second_order_quad_faces : 0
n_tet_cells : 0
n_pyra_cells : 0
n_prism_cells : 0
n_poly_cells : 0
n_hex_cells : 0
n_second_order_tet_cells : 0
n_second_order_pyra_cells : 0
n_second_order_prism_cells : 0
n_second_order_hex_cells : 0
n_unmeshed_topo_faces : 0
/opt/hostedtoolcache/Python/3.12.11/x64/lib/python3.12/site-packages/ansys/meshing/prime/graphics/plotter.py:362: UserWarning: DeprecationWarning: The `Graphics` class is deprecated. Use the `PrimePlotter` class instead.
warnings.warn(
Mesh generation#
The mesh is generated using the Ansys PRIME API. The mesh is generated using the following steps:
Initialize the connection tolerance and other parameters.
Scaffold the part.
Mesh the surfaces.
Write the mesh to a file.
# Initialize the connection tolerance and other parameters --
#
# Target element size
element_size = 0.5
# Initialize the parameters
params = prime.ScaffolderParams(
model,
absolute_dist_tol=0.1 * element_size,
intersection_control_mask=prime.IntersectionMask.FACEFACEANDEDGEEDGE,
constant_mesh_size=element_size,
)
# Get existing topoface or topoedge IDs
faces = part.get_topo_faces()
beams = []
scaffold_res = prime.Scaffolder(model, part.id).scaffold_topo_faces_and_beams(
topo_faces=faces, topo_beams=beams, params=params
)
print(scaffold_res)
n_incomplete_topo_faces : 0
error_code : 0
Surface meshing#
The surface mesh is generated using the previous element size and the topological faces of the part.
surfer_params = prime.SurferParams(
model=model,
size_field_type=prime.SizeFieldType.CONSTANT,
constant_size=element_size,
generate_quads=True,
)
surfer_result = prime.Surfer(model).mesh_topo_faces(part.id, topo_faces=faces, params=surfer_params)
# Display the mesh
if GRAPHICS_BOOL:
display = Graphics(model=model)
display()
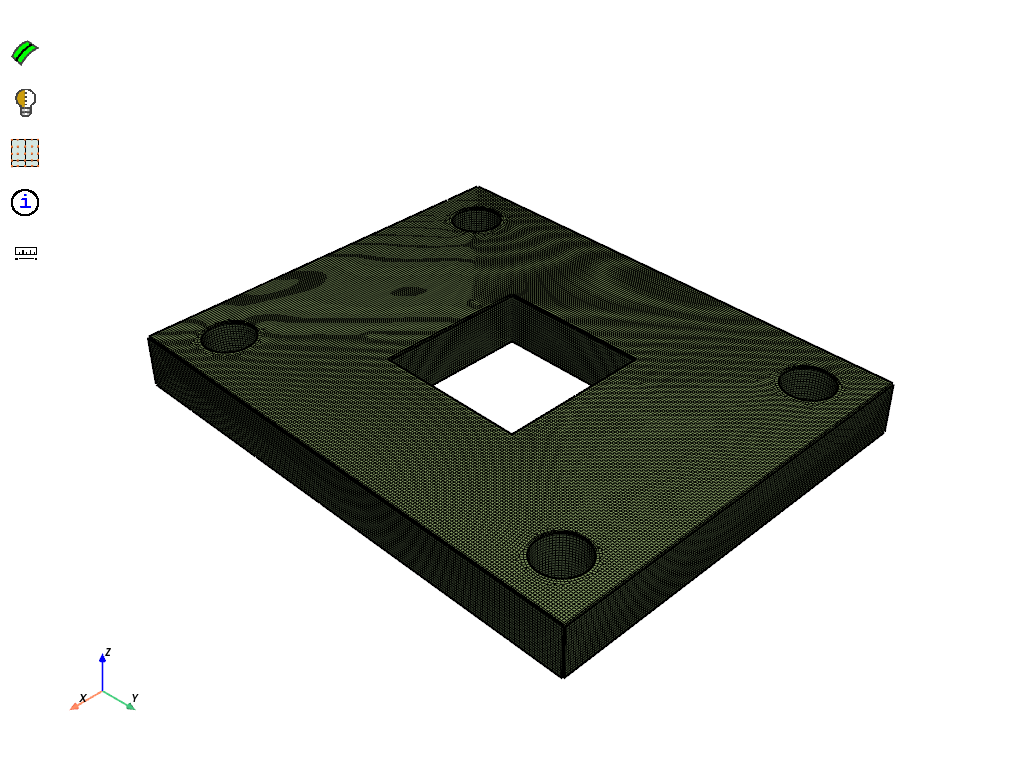
/opt/hostedtoolcache/Python/3.12.11/x64/lib/python3.12/site-packages/ansys/meshing/prime/graphics/plotter.py:362: UserWarning: DeprecationWarning: The `Graphics` class is deprecated. Use the `PrimePlotter` class instead.
warnings.warn(
Export the mesh#
The mesh is exported to a CDB file. The CDB file can be used to create a MAPDL case.
mapdl_cdb = Path(OUTPUT_DIR, "modeling_demo.cdb")
file_io.export_mapdl_cdb(mapdl_cdb, params=prime.ExportMapdlCdbParams(model))
assert os.path.exists(mapdl_cdb)
print(f"MAPDL case exported at {mapdl_cdb}")
This get_abaqus_simulation_data is a beta API. The behavior and implementation may change in future.
MAPDL case exported at /home/runner/work/pyansys-workflows/pyansys-workflows/geometry-mesh/outputs/modeling_demo.cdb
Close the PRIME session#
Close the PRIME session to release the resources. This is important to prevent memory leaks.
prime_client.exit()
Total running time of the script: (0 minutes 27.790 seconds)